As a PHP developer, it is crucial to follow coding guidelines and recommended practices to ensure code quality, maintainability, and security.
By adhering to these practices, you can streamline the development process, improve code performance and reliability, and reduce the likelihood of errors and security vulnerabilities. Moreover, following consistent coding standards will make your code more understandable and accessible to other developers, and documentation can ensure the smooth collaboration and maintenance of larger projects.
Whether you are a beginner or an experienced PHP developer, this guide will provide valuable insights and recommendations to help you enhance your coding skills and develop high-quality PHP projects. So, let’s dive in and explore the best practices for PHP coding!
PHP Coding Standards
PHP coding standards play a crucial role in developing high-quality and maintainable code. Coding standards are a set of guidelines that developers follow to ensure code consistency and readability. Adhering to coding standards not only makes it easier for other developers to understand your code but also improves your code quality.
Popular coding standards in PHP include PSR-1 and PSR-2, which were established by the PHP community. PSR stands for PHP Standard Recommendations, and they provide standards for basic coding styles like class names, file naming, and function arguments. Adhering to these standards helps ensure that your code is uniform and accessible to other developers.
By following coding standards, you improve the readability and maintainability of your code. It makes it easier to modify and debug your code in the future. Additionally, following PHP coding standards can help you avoid common coding mistakes and improve the performance of your application.
Why are Coding Standards Important in PHP Development?
PHP coding standards are important because they help you write high-quality code that is easy to read, maintain, and debug. They ensure that your code is consistent, which is important when you have multiple developers working on the same project. It makes it easier for others to understand and work with your code.
Coding standards also help ensure that your code is error-free and secure. They can prevent common coding mistakes and vulnerabilities that could lead to security breaches. By adhering to coding standards, you can avoid wasting time on code reviews or bug fixes, allowing you to focus on developing new features.
Overall, PHP coding standards are essential to developing high-quality code that is easy to read, maintain, and secure.
Proper Error Handling
Errors are an inevitable part of PHP development, and handling them correctly is crucial to developing robust and reliable applications. Proper error handling can help developers identify and fix issues quickly, minimizing the impact on end-users.
One recommended practice for handling errors in PHP is to use try-catch blocks. This allows developers to catch and handle exceptions in a controlled manner. For example:
try {
//code that may throw an exception
} catch(Exception $e) {
//handle the exception
}
Another recommended practice is to log errors to a file or database. This can help developers track errors and identify patterns or trends that could indicate deeper issues. For example:
try {
//code that may throw an exception
} catch(Exception $e) {
//log the exception
error_log($e->getMessage(), 3, "/var/log/my-errors.log");
}
It is important to note that while error logging can be helpful, sensitive information should not be logged, as it could compromise the security of the application.
By following recommended practices for error handling in PHP development, developers can improve the reliability and security of their code, and deliver a better experience for end-users.
Secure Coding Practices
When it comes to PHP development, incorporating security measures is critical to prevent potential vulnerabilities. Hackers are constantly seeking out these vulnerabilities to exploit to gain unauthorized access to data and systems. As such, it’s important to adhere to recommended practices for PHP coding to shore up your code’s defenses against these security threats.
One such practice is input validation. By ensuring that user input is validated before being used, you can better protect against SQL injections and other types of attacks.
Another recommended practice is utilizing prepared statements. Prepared statements allow developers to parameterize database queries, which can help to prevent SQL injection attacks by separating the query logic from the user input.
Additionally, it’s important to follow proper authentication and authorization protocols. Implementing authentication and access controls can help to ensure that only authorized users are accessing protected resources.
Recommended Practices for Secure PHP Coding |
---|
Perform input validation on all user input |
Use prepared statements to separate user input from query logic |
Implement proper authentication and access controls |
Keep your PHP version up to date to access security patches |
Staying up to date with PHP’s latest security patches is also important. New vulnerabilities are regularly discovered, and PHP developers must make sure their code is written in compliance with the latest security updates to mitigate risks.
By adhering to recommended practices for PHP coding and utilizing secure coding practices, you can greatly improve the security of your PHP applications, mitigating the risk of data breaches and unauthorized access to your systems and data.
Optimizing PHP Code
Optimizing PHP code is an important aspect of PHP development. The performance of a PHP application can be greatly enhanced by optimizing the code. Here are some recommended practices that can assist in optimizing PHP code:
Code Profiling
Code profiling is the process of analyzing an application’s performance by measuring the time it takes to execute code. This process can help developers determine which parts of the code are taking the most time to execute and optimize them accordingly. There are several code profiling tools available for PHP developers, such as Xdebug, Blackfire, and PHP Debug Bar.
Caching
Caching is the process of storing data in a temporary location to reduce the loading time of a web page. In PHP, caching can be achieved by using caching extensions such as APC, Memcached, or Redis. These tools store data in memory and reduce the time it takes to retrieve data from a database or file system.
Minimizing Database Queries
Reducing the number of database queries can greatly improve the performance of a PHP application. Developers can optimize queries by using indexes, avoiding subqueries, and reducing the amount of data retrieved from the database. Additionally, using an Object-Relational Mapping (ORM) tool like Doctrine or Propel can help minimize database queries by abstracting the database access layer.
By following these recommended practices, PHP developers can significantly improve the performance of their applications. With code profiling, caching, and minimizing database queries, PHP applications can run faster and be more responsive to user requests.
Testing and Debugging Strategies
Testing and debugging are essential components of PHP development. By testing code regularly, developers can identify and fix issues early on, avoiding potential problems down the line. Proper debugging techniques can also help streamline the development process, saving time and effort in the long run.
There are several testing methodologies that PHP developers can use, such as unit testing, integration testing, and acceptance testing. Unit testing involves testing individual functions or components of code in isolation, while integration testing involves testing how those components work together. Acceptance testing involves testing the entire application to ensure it meets the requirements set forth by the client or end-user.
When it comes to debugging, there are several tips and tools that can help developers identify and fix issues quickly. One useful tool is the PHP debugging extension Xdebug, which can be used to step through code and trace where errors occur. Another helpful technique is to use logging to record events and messages during the execution of code, providing a useful trail of information for debugging purposes.
Debugging Tips:
- Use Xdebug to step through code and trace errors
- Use logging to record events and messages during code execution
- Use print statements to output variable values and other information for easier debugging
By incorporating testing and debugging strategies into their development process, PHP developers can ensure that their code is both functional and high-quality. This can lead to a better end-user experience and make maintenance and updates easier in the future.
Version Control and Collaborative Development
Version control systems (VCS) are an essential tool for PHP developers to manage code changes and collaborate effectively. Version control systems like Git, SVN, and Mercurial help ensure that the codebase is always up-to-date and easily accessible to all team members.
Collaborative development is another important aspect of PHP development. With the rise of distributed teams and remote work, developers must be equipped with the tools and practices to work effectively together. Tools like GitHub and Bitbucket provide an excellent platform for developers to collaborate on code changes, manage issues and bugs, and review each other’s work.
Version Control Workflows
There are several version control workflows that PHP developers can adopt, such as the Gitflow workflow, Feature Branch workflow, and Centralized workflow. These workflows help keep the codebase organized and maintainable.
The Gitflow workflow is a popular approach that involves creating two branches – the master branch and the develop branch. All changes to the codebase are made to the develop branch, and once the changes are tested and approved, they are merged into the master branch. This workflow ensures that the master branch always has stable and releasable code.
The Feature Branch workflow is another approach that involves creating a new branch for each feature or task. This approach enables developers to work on different tasks simultaneously without interfering with each other’s code. Once the feature is complete, it can be merged into the main branch.
The Centralized workflow is simpler and involves a single main branch where all changes are made. This approach is suitable for smaller teams or projects with less frequent code changes.
Whichever workflow you choose, it’s important to ensure that every team member adheres to it for consistency and maintainability.
Collaborative development using version control systems is an essential best practice for PHP development. Effective collaboration helps developers work together to deliver high-quality code that is stable, reliable, and maintainable.
Documentation and Commenting
When it comes to PHP development, documentation and meaningful comments are crucial to ensure that code is maintainable and easily understandable. Not only does it improve code comprehension, but it also facilitates collaboration and promotes knowledge sharing among team members.
Experts recommend using documentation tools and following standards for consistent formatting. By taking the time to document code thoroughly and leaving comments that explain complex processes, developers can save time and reduce confusion in the long run.
One recommended practice is to start each file with a comment header that explains the purpose of the file, the author, and the date of creation. Comments should also be used to explain tricky code blocks and to provide insight into the logic behind the code.
It’s important to keep in mind that documentation and commenting should be considered an ongoing process. As code evolves, so should its documentation. A good rule of thumb is to update comments whenever changes are made to the code.
To ensure that code remains readable and maintainable, it’s important to make a concerted effort to document and comment throughout the development process. Doing so promotes consistency and makes it easier to onboard new team members.
External Resources
FAQ
Q: What are the benefits of following best practices in PHP development?
A: Following best practices in PHP development can improve code readability, maintainability, and security. It can also enhance collaboration among developers and make the code easier to debug and optimize.
Q: What are some popular coding standards in PHP development?
A: Some popular coding standards in PHP development include PSR-1 and PSR-2. These standards provide guidelines on coding style, naming conventions, and file organization.
Q: Why is proper error handling important in PHP development?
A: Proper error handling is important in PHP development to ensure that errors are handled gracefully and do not affect the stability or security of the application. It helps in debugging and troubleshooting issues and provides a better user experience.
Q: What are some secure coding practices in PHP development?
A: Some secure coding practices in PHP development include input validation, using prepared statements to prevent SQL injection, and sanitizing user input before processing. These practices help in mitigating common security vulnerabilities.
Q: How can PHP code be optimized for better performance?
A: PHP code can be optimized for better performance by using techniques such as code profiling to identify bottlenecks, caching to reduce database queries, and optimizing algorithms and data structures. These optimizations can significantly improve the speed and efficiency of the application.
Q: Why is testing and debugging important in PHP development?
A: Testing and debugging are important in PHP development to ensure that the code functions as expected and to identify and fix any issues or bugs. Testing methodologies such as unit testing and integration testing help in validating the functionality of the code, while debugging tools assist in identifying and resolving errors.
Q: What are the benefits of using version control systems in PHP development?
A: Using version control systems in PHP development provides benefits such as tracking changes made to the code, facilitating collaboration among multiple developers, and allowing easy rollback to previous versions. It also helps in maintaining a history of changes and resolving conflicts.
Q: Why is documentation and commenting important in PHP development?
A: Documentation and commenting are important in PHP development to make the code more understandable and maintainable. They provide information about the purpose and functionality of the code, making it easier for other developers to work with and modify the code.
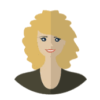
Ashley is an esteemed technical author specializing in scientific computer science. With a distinguished background as a developer and team manager at Deloit and Cognizant Group, they have showcased exceptional leadership skills and technical expertise in delivering successful projects.
As a technical author, Ashley remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Their expertise in PHP web development, coupled with their experience as a developer and team manager, positions them as a valuable resource for professionals seeking guidance and best practices. With each publication, Ashley strives to empower readers, inspire creativity, and propel the field of scientific computer science forward.