If you’re a PHP developer, you know how important it is to have a streamlined development workflow. Docker can help simplify that process. Docker is an open-source platform that allows you to run applications in isolated containers, making it easier to develop, deploy, and manage your PHP applications.
Docker and its Concepts
If you’re a PHP developer looking to streamline your development workflow, Docker can be an invaluable tool. Docker is a containerization platform that allows you to package an application and its dependencies into a single container, making it portable and easy to deploy across different environments.
Using Docker with PHP development has several benefits, including:
- Consistent environments: With Docker, you can ensure that your development environment is the same as your production environment, reducing the likelihood of bugs and issues arising in deployment.
- Isolation: Containers provide a level of isolation between applications, so you can run multiple PHP applications on the same server without worrying about them interfering with each other.
- Manageability: Docker makes it easy to manage dependencies and versions of different libraries and packages necessary for PHP development.
Containers, Images, and Dockerfile: Core Concepts of Docker
To understand how Docker fits into PHP development, let’s dive into the core concepts of Docker: containers, images, and Dockerfile.
A container is a lightweight, standalone, and executable software package that contains everything an application needs to run, including code, libraries, and dependencies. An image, on the other hand, is a read-only template that defines the container’s filesystem and configuration.
A Dockerfile is a file that contains a set of instructions for building a Docker image. It typically contains commands for installing dependencies, copying files, and exposing ports.
When you run a Docker container, you are essentially creating an instance of an image. Multiple containers can be created from the same image, with each container running in its own isolated environment.
Using Docker with PHP development involves creating a Docker image for your PHP application and its dependencies, which can then be run in a container. This allows you to easily package and deploy your PHP application across different environments without having to worry about version conflicts or differences in system configurations.
Setting Up Docker for PHP Development
Using Docker with PHP development can greatly simplify the development process and environment setup. Here is a step-by-step guide on how to set up Docker for PHP development:
Step 1: Install Docker
The first step is to install Docker on your machine. You can download the installer from the official Docker website and follow the installation instructions for your operating system.
Step 2: Create a Dockerfile
Once you have Docker installed, create a Dockerfile to define the environment for your PHP application. This file specifies the base image, adds any necessary dependencies, and sets up the application environment.
Step 3: Build the Docker Image
After creating the Dockerfile, you can build the Docker image by running the following command in your terminal:
docker build -t my-php-app .
This will create a Docker image based on the Dockerfile and tag it as “my-php-app”.
Step 4: Start a Container
Now that you have a Docker image, you can create a container to run your PHP application. To do this, run the following command:
docker run -p 8080:80 my-php-app
This will start a container based on the “my-php-app” image and map port 8080 on your machine to port 80 in the container.
Step 5: Test the Application
You can now test your PHP application by opening a web browser and navigating to http://localhost:8080/. Your application should be up and running!
This is just a basic setup, but you can customize your PHP development environment with Docker to suit your specific needs.
Dockerizing PHP Applications
Dockerizing PHP applications involves creating Docker images that contain all the necessary components to run the application. This allows for easy deployment and reduces the risk of errors or compatibility issues. Here’s how to Dockerize a PHP application:
1. Define your Dockerfile
The Dockerfile is a script that defines all the necessary components for the Docker image. This includes the base image, environment variables, and any dependencies required for the PHP application to run. Here’s an example Dockerfile for a Laravel application:
FROM php:7.4
WORKDIR /app
COPY . /app
RUN php artisan optimize
ENTRYPOINT ["php", "artisan", "serve"]
2. Build your Docker image
Once the Dockerfile is defined, you can build the Docker image by running the following command in your project directory:
$ docker build -t my-php-app:latest .
This will create a Docker image with the tag “my-php-app:latest” using the Dockerfile in the current directory.
3. Run your Docker container
With the Docker image created, you can run your PHP application in a Docker container using the following command:
$ docker run -p 8000:8000 my-php-app
This will start a Docker container with the name “my-php-app” and map port 8000 on the host machine to port 8000 in the container. You can access your PHP application by navigating to http://localhost:8000 in your web browser.
4. Push your Docker image to a registry
Once you have successfully Dockerized your PHP application, you can push the Docker image to a registry such as Docker Hub. This will allow for easy deployment to other machines or servers. Here’s an example command for pushing your Docker image:
$ docker push my-php-app:latest
This will push the Docker image with the tag “my-php-app:latest” to Docker Hub.
Dockerizing PHP applications can greatly simplify the deployment process and ensure compatibility across environments. By following these steps, you can quickly and easily Dockerize your PHP applications.
Managing Dependencies with Docker
Managing dependencies is a crucial part of PHP development. Docker can help streamline this process by allowing developers to create containerized environments for managing different versions of PHP and third-party libraries.
Using Docker with PHP development allows developers to isolate their dependencies, which helps avoid conflicts and compatibility issues. It also provides a consistent development environment, making it easier to reproduce issues and ensure that code runs consistently across different machines.
Dockerizing PHP applications is a simple process that involves creating a Dockerfile that specifies the application’s dependencies and configuration. Docker then builds an image from the Dockerfile, which can be used to run the application in a container.
By using Docker to manage dependencies, developers can also easily switch between different versions of PHP and third-party libraries without having to modify their local environment. This makes it easier to test and develop applications in different environments and speeds up the development process overall.
Dockerizing Third-Party Libraries
Docker can also be used to containerize third-party libraries, making it easier to manage dependencies across multiple projects. Developers can create separate containers for different libraries, which can be easily shared across different projects and environments.
For example, a developer might create a Docker container for a specific version of a database engine or a particular PHP extension. This container can be easily shared with other developers who are working on projects that require the same dependencies.
Dockerizing third-party libraries also allows developers to easily update dependencies without affecting other projects. They can simply update the container image and deploy the updated version to all projects that require the updated library.
Streamlining Development with Docker Compose
Docker can greatly enhance the development workflow for PHP applications. However, managing multi-container environments manually can be time-consuming and error-prone. This is where Docker Compose comes in, providing a way to define, manage, and orchestrate multi-container setups.
What is Docker Compose?
Docker Compose is a tool that allows you to manage multiple containers as a single application. It uses a YAML file to define the configuration of all the containers that make up your application, along with the networking and volume configurations required for them to communicate with one another.
How can Docker Compose improve your workflow?
With Docker Compose, you can define your entire application stack as code and easily spin up and tear down your environments. This helps ensure consistency and reproducibility, making it easy to share your development environment with colleagues or move your application to production.
How to get started with Docker Compose?
To get started with Docker Compose, you’ll need to write a YAML file that defines your application stack. This file specifies the different services that make up your application, along with networking and volume configurations. Once you have your YAML file ready, you can use the docker-compose
command-line tool to start or stop your application stack.
Here’s an example YAML file that defines a simple PHP application stack:
Service | Description |
---|---|
web | Runs an Apache web server with PHP support |
db | Runs a MySQL database server |
```
version: '3'
services:
web:
build: .
ports:
- "80:80"
links:
- db
db:
image: mysql
environment:
MYSQL_ROOT_PASSWORD: example
```
In this example, we’re defining two services: web
and db
. The web
service is defined by building an image from the .
directory and exposing port 80 for HTTP traffic. We also link the web
service to the db
service so that they can communicate with each other. The db
service is defined using the standard MySQL Docker image and sets the database root password.
Once you have your YAML file, you can start your application stack by running the following command:
docker-compose up
This will start all the containers defined in your YAML file and link them together as specified. You can then access your PHP application at http://localhost
.
In conclusion, Docker Compose can greatly simplify the process of managing multi-container environments for PHP applications. By defining your application stack as code, you can easily spin up and tear down your environments, ensuring consistency and reproducibility throughout your development workflow.
Testing PHP Applications with Docker
Using Docker with PHP development provides a range of benefits, including better control over dependencies and the ability to streamline development workflows. Docker can also simplify the testing process, allowing developers to create consistent test environments that can be easily reproduced.
With Docker, testing PHP applications becomes more reliable and efficient. Developers can create test environments that can be replicated across different machines and operating systems, ensuring that tests produce consistent results. Moreover, using Docker to manage test environments means that developers can easily test different configurations of their applications and ensure that they’re compatible with various dependencies.
To set up a test environment using Docker, developers can use a Dockerfile to define the environment’s configuration and dependencies. They can then use Docker Compose to create and manage multi-container environments for running tests. This approach lets developers define different services for testing, such as databases or other third-party services, ensuring that the environment can be easily reproduced.
Another benefit of using Docker for testing is that it allows developers to run tests in isolation. Developers can create containerized test environments and run tests within these containers without affecting the host machine. This approach helps avoid conflicts between different versions of PHP or third-party libraries that may be installed on the host machine.
In summary, using Docker with PHP development can significantly improve the testing process and streamline workflows. By providing a consistent and easily reproducible environment for testing, developers can reduce errors and ensure that their applications work as intended. As Docker continues to gain popularity among PHP developers, it’s becoming an essential tool for anyone looking to optimize their development workflow and simplify testing processes.
Deploying Dockerized PHP Applications
Once you have Dockerized your PHP application, the next step is to deploy it. There are multiple options for deploying Dockerized PHP applications, depending on your specific needs.
Deployment Strategies:
There are various deployment strategies for Dockerized PHP applications, such as:
- Deploying to a single server using Docker Compose
- Deploying to multiple servers using Kubernetes
- Using a cloud-based container service such as AWS Elastic Container Service or Google Cloud Platform Kubernetes Engine
Container Orchestration Tools:
Container orchestration tools, such as Kubernetes and Docker Swarm, can manage and automate the deployment of containers. These tools can also provide load balancing and scaling capabilities to ensure your PHP application can handle high traffic loads.
Best Practices:
When deploying Dockerized PHP applications, there are some best practices to keep in mind:
- Use a production-ready Docker image
- Use a separate container for your database
- Set up proper environment variables and secrets
- Use a reverse proxy for SSL termination and load balancing
By following these best practices and utilizing the appropriate deployment strategy and container orchestration tool, you can confidently deploy your Dockerized PHP application for smooth and reliable operation.
Docker is a valuable tool for PHP developers. Docker benefits for PHP devs include streamlining workflows, managing dependencies, and simplifying deployment. By Dockerizing PHP apps, developers can create containerized environments that are consistent and reproducible.
Throughout this article, we have explored the different aspects of using Docker with PHP development. We have covered the core concepts of Docker, how to set up Docker for PHP development, and how to Dockerize PHP applications. We have also looked at managing dependencies with Docker and streamlining development with Docker Compose. Finally, we discussed how Docker can simplify the process of testing and deploying PHP applications.
By incorporating Docker into their PHP development workflow, developers can save time and reduce the risk of errors. And with the popularity of containerization growing rapidly, the knowledge and skills gained from working with Docker can be extremely valuable in the job market.
So, whether you are a seasoned PHP developer or just starting out, Docker is a tool that you should definitely consider using. By following the instructions and examples provided in this article, you can get started with Dockerizing your PHP apps today.
External Resources
FAQ
Q: What are the benefits of using Docker for PHP developers?
A: Docker allows PHP developers to streamline their development workflow by providing a consistent and reproducible environment. It eliminates the need for manual setup and configuration, making it easier to onboard new team members and collaborate on projects.
Q: How does Docker benefit PHP developers?
A: Docker provides isolation for PHP applications, allowing developers to package their code and dependencies into containers. This isolation ensures that the application runs consistently across different environments, from development to production.
Q: What does it mean to Dockerize a PHP application?
A: Dockerizing a PHP application involves creating a Docker image that contains the necessary dependencies and configurations for running the application. This image can then be used to launch containers that run the PHP application.
Q: Can Docker help with managing dependencies in PHP development?
A: Yes, Docker can help manage dependencies in PHP development. By containerizing different versions of PHP and third-party libraries, developers can easily switch between different dependency configurations without conflicts.
Q: What is Docker Compose and how does it help with PHP development?
A: Docker Compose is a tool that allows developers to define and manage multi-container environments. In PHP development, Docker Compose can be used to set up the entire development environment, including web servers, databases, and other services.
Q: How can Docker simplify the testing process for PHP applications?
A: Docker provides a consistent and isolated environment for testing PHP applications. Test environments can be easily created using Docker, ensuring that the application is tested in the same environment as it will run in production.
Q: What are some options for deploying Dockerized PHP applications?
A: There are multiple options for deploying Dockerized PHP applications, including using container orchestration tools like Kubernetes or deploying to cloud platforms that support Docker, such as AWS or Google Cloud.
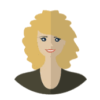
Ashley is an esteemed technical author specializing in scientific computer science. With a distinguished background as a developer and team manager at Deloit and Cognizant Group, they have showcased exceptional leadership skills and technical expertise in delivering successful projects.
As a technical author, Ashley remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Their expertise in PHP web development, coupled with their experience as a developer and team manager, positions them as a valuable resource for professionals seeking guidance and best practices. With each publication, Ashley strives to empower readers, inspire creativity, and propel the field of scientific computer science forward.