As you know, email is a crucial communication tool in web development, and PHP offers a built-in mail function to send emails programmatically. However, the traditional PHP mail function has limitations, such as poor deliverability, security vulnerabilities, and lack of customization options.
That’s why it’s crucial to explore alternative communication methods to optimize email communication in PHP.
Exploring Alternative Communication Methods
PHP Mail is a simple function for sending emails in PHP, but it has its limitations. For instance, it cannot handle large attachments and frequently ends up in spam folders. Fortunately, there are several alternatives to PHP Mail that can improve the security and reliability of email communication in PHP.
Third-Party Email Libraries
One popular alternative to PHP Mail is using third-party email libraries that provide a more robust email interface. These libraries offer features like email templating, file attachments, and HTML formatting. Two notable examples of these libraries are PHPMailer and SwiftMailer.
Library | Pros | Cons |
---|---|---|
PHPMailer | Easy to integrate with PHP. Requires minimum configuration. Supports many email protocols. | Not as feature-rich as other libraries. |
SwiftMailer | Flexible and customizable. Offers more features than PHPMailer. Great for large email campaigns. | Requires more setup and configuration. Can be challenging for beginners. |
When choosing between these libraries, consider your specific needs and the level of customization required.
SMTP Servers for Email Delivery
Another alternative to PHP Mail is using an SMTP server to send emails. SMTP servers are designed for high-volume email delivery and can improve the reliability and deliverability of emails. Some popular SMTP servers include SendGrid, Amazon SES, and Mailgun.
SMTP servers require more configuration than third-party email libraries, but they offer better scalability and control over email communication.
API Integrations for Email Communication
API integrations are another alternative to PHP Mail, allowing developers to send emails directly from their applications via an API endpoint. This option offers greater customization and functionality than other alternatives. Some popular email API services include Twilio SendGrid, Mailgun, and Postmark.
API integrations require more coding and configuration than other alternatives, but they can provide greater control over email communication and allow for more granular error handling.
Overall, the best alternative to PHP Mail depends on the specific needs of your application. Consider the level of customization required, the volume of emails being sent, and the level of control needed over email communication.
- Looking for more information on alternatives to PHP Mail? Check out our Third-Party Email Libraries Comparison Guide.
- Not sure which SMTP server to use? Our SMTP Server Comparison Guide can help.
- Want to learn more about integrating email APIs? Check out our article on Getting Started With Email APIs in PHP.
Third-Party Email Libraries
There are several popular third-party email libraries that offer an alternative to the traditional PHP mail function. These libraries provide advanced features, such as support for attachments, HTML emails, and SMTP authentication, which are not available in the built-in mail() function.
One of the most widely used email libraries is PHPMailer. PHPMailer is a full-featured email creation and transfer class for PHP that offers extensive functionality for sending email messages. It supports SMTP authentication, HTML and plain text emails, file attachments, and more. Additionally, PHPMailer is easy to use and integrates seamlessly with popular PHP frameworks, such as Laravel and CodeIgniter.
Another popular email library is SwiftMailer. SwiftMailer is a flexible and powerful email system that provides a rich set of features for creating and sending email messages. It supports various transport methods, including SMTP, sendmail, and Amazon SES. It also supports HTML emails, file attachments, and SMTP authentication. Additionally, SwiftMailer is highly customizable and extensible, making it a great choice for more complex email requirements.
PHPMailer vs. SwiftMailer: Which One to Choose?
Both PHPMailer and SwiftMailer are excellent choices for sending emails in PHP. They offer similar features and functionality, with some differences in terms of implementation and design. Ultimately, the choice between the two depends on your specific needs and preferences.
PHPMailer is generally considered easier to use and has a more straightforward API. It also has good integration capabilities with popular PHP frameworks. However, some users have reported issues with compatibility and security vulnerabilities in the past.
SwiftMailer, on the other hand, has a more complex API and may require more configuration to get started. However, it offers greater customization options and is more extensible, making it a better choice for more advanced email requirements. It also has a good reputation for security and stability.
When choosing between these two libraries, it is important to consider factors such as ease of use, level of customization, and security. It may be helpful to try out both libraries and see which one works best for your specific use case.
SMTP Servers for Email Delivery
SMTP servers offer a reliable and efficient alternative to the traditional PHP mail function. By utilizing an SMTP server, you can improve your email deliverability and ensure that your messages are not marked as spam. SMTP servers work by establishing a connection with the recipient’s email server and delivering the message directly, instead of relying on the PHP mail function to send the email.
One popular SMTP server option is Mailgun. It offers features such as email tracking, analytics, and the ability to configure custom domains.
Another option is SendGrid, which offers a user-friendly API and real-time analytics. Both Mailgun and SendGrid offer free tier plans with limited usage, making them affordable for small businesses or personal projects.
How to Use an SMTP Server in PHP
To utilize an SMTP server for email delivery in PHP, you will need to use an email library that supports SMTP. PHPMailer and SwiftMailer are both libraries that support SMTP and offer many features beyond just email delivery. Here is an example of how to use PHPMailer to send an email using an SMTP server:
// Load PHPMailer library require_once 'phpmailer/PHPMailerAutoload.php'; // Create a new PHPMailer instance $mail = new PHPMailer; // Tell PHPMailer to use SMTP $mail->isSMTP(); // Configure the SMTP server details $mail->Host = 'smtp.example.com'; $mail->SMTPAuth = true; $mail->Username = 'yourusername'; $mail->Password = 'yourpassword'; $mail->SMTPSecure = 'tls'; $mail->Port = 587; // Set the email details $mail->setFrom('your@email.com', 'Your Name'); $mail->addAddress('recipient@email.com', 'Recipient Name'); $mail->Subject = 'Email Subject'; $mail->Body = 'Email message body'; // Send the email if (!$mail->send()) { echo 'Message could not be sent.'); echo 'Mailer Error: ' . $mail->ErrorInfo; } else { echo 'Message has been sent.'; }
By using an SMTP server with PHPMailer or SwiftMailer, you can take control of your email delivery and ensure that your messages are delivered reliably and securely.
API Integrations for Email Communication
If you’re looking for a more flexible and feature-rich approach to email communication in PHP, API integrations might be the answer. By using third-party API services, you can customize your email communication to fit your specific needs and gain access to additional functionality.
One popular email API service is SendGrid, which offers powerful tools for email marketing, transactional emails, and email analytics. By integrating SendGrid into your PHP application, you can send emails directly through their servers and take advantage of features like template customization, recipient management, and delivery optimization.
Another option is Mailgun, which provides a similar suite of email automation tools and performance tracking. With Mailgun, you can also set up webhooks to receive notifications about specific email events, such as opens and clicks, and trigger custom actions in your PHP application.
Implementation Example: SendGrid API
Here’s an example of how to use the SendGrid API in PHP to send an email:
// Include the SendGrid library
require './sendgrid-php/sendgrid-php.php';
// Create a new SendGrid object
$sendgrid = new \SendGrid('YOUR_API_KEY');
// Define the email message
$email = new \SendGrid\Mail\Mail();
$email->setFrom("from@example.com", "Sender Name");
$email->setSubject("Email Subject");
$email->addTo("to@example.com", "Recipient Name");
$email->addContent("text/plain", "Email Content");
// Send the email using the SendGrid API
try {
$response = $sendgrid->send($email);
echo "Email sent successfully!";
} catch (Exception $e) {
echo 'Caught exception: ', $e->getMessage(), "\n";
}
This code uses the SendGrid PHP library to create a new email message and send it through the SendGrid API. You’ll need to provide your own API key and define the email address and content fields based on your specific use case.
API integrations offer a lot of flexibility and customization options, but they also require more setup and API knowledge than other email communication methods. It’s important to choose an API service that fits your needs and budget, and carefully follow the documentation and best practices to ensure secure and reliable email delivery.
Comparison: PHPMailer vs. SwiftMailer
When it comes to choosing a third-party email library, there are many options available. Two of the most popular ones are PHPMailer and SwiftMailer.
PHPMailer:
Feature | Description |
---|---|
Easy to use | PHPMailer provides a simple and intuitive interface that makes it easy to send emails in PHP. |
Supports multiple email formats | PHPMailer allows you to send emails in various formats, including HTML, plain text, and attachments. |
Integration capabilities | PHPMailer can be easily integrated with SMTP servers, such as Gmail or Yahoo, to ensure reliable and secure email delivery. |
SwiftMailer:
Feature | Description |
---|---|
Flexible and customizable | SwiftMailer provides a wide range of features and options, allowing you to customize your email templates and delivery settings. |
Efficient email handling | SwiftMailer is designed to handle large volumes of emails efficiently, ensuring that your messages are delivered quickly and reliably. |
Supports multiple protocols | SwiftMailer supports not only SMTP, but also Sendmail and other popular email protocols. |
Both PHPMailer and SwiftMailer are excellent choices for sending emails in PHP, and the decision ultimately comes down to personal preference and specific project requirements. However, by using either of these libraries, you can ensure that your email communication in PHP is efficient, secure, and reliable.
Implementation for Alternative Communication Methods
When implementing alternative communication methods in PHP, it’s crucial to consider several factors to ensure efficient and secure email delivery.
Security Measures
One of the most critical aspects to bear in mind is security. With the growing number of cyber threats, using a secure email delivery method is essential to protect sensitive information. Therefore, it’s recommended to use encrypted connections, such as SSL or TLS, and authenticate the sender to avoid spamming or phishing attacks.
Error Handling
Another important consideration is error handling. While sending emails, it’s common to encounter errors that can lead to failed deliveries or unexpected results. Therefore, it’s vital to handle errors proactively, log them properly, and provide appropriate feedback to users to resolve issues in a timely manner.
Configuration Options
Configuring alternative communication methods can be quite daunting, especially for beginners. Therefore, it’s essential to provide configuration options that are easy to understand and modify. It’s also advisable to use a configuration file instead of hard-coding options in the code to simplify maintenance and avoid errors.
Scalability
As organizations grow, the volume of emails also increases, making scalability a crucial concern. Therefore, it’s essential to choose a communication method that can scale with the company’s growth. For instance, SMTP servers are highly scalable because they’re designed to handle large volumes of emails efficiently.
By considering these implementation factors, organizations can optimize email communication in PHP and ensure efficient and secure email delivery.
Optimizing Email Communication in PHP
Efficient and secure communication methods are crucial in PHP development, especially when it comes to email communication. However, the traditional PHP mail function may not always meet the desired standards. It is important to explore alternatives that can improve email deliverability, customization, and functionality.
Third-party email libraries like PHPMailer and SwiftMailer provide a user-friendly and feature-rich approach to sending emails in PHP. SMTP servers, on the other hand, offer reliable delivery and better handling of email errors. API integrations provide a way to leverage third-party email services and customize email functionality to meet specific needs.
When considering implementing alternative communication methods, it is important to take into account several implementation considerations. These include ensuring proper security measures, handling errors and exceptions, configuring options for maximum efficiency, and scalability.
Real-world examples of companies and industries that have successfully implemented alternative communication methods in PHP show the benefits of exploring different options. Keeping up with the latest PHP email communication options and industry trends can help developers stay ahead of the curve and improve their email communication processes.
Summary
Optimizing email communication in PHP is crucial for efficient and secure communication processes. Third-party email libraries, SMTP servers, and API integrations are all viable alternatives to the traditional PHP mail function. Factors such as security, error handling, configuration, and scalability should be taken into consideration when implementing alternative methods. Staying informed about emerging trends and successful implementations can help developers improve email communication processes.
- Explore alternative communication methods to improve email deliverability, customization, and functionality in PHP development.
- Implement best practices for ensuring security, handling errors and exceptions, configuring options, and scalability.
- Stay informed about emerging trends and successful implementations for continuous improvement.
External Resources
https://github.com/PHPMailer/PHPMailer
https://swiftmailer.symfony.com/
FAQ
Frequently asked questions.
If you have anything else you want to ask, email us.
Here are five frequently asked questions about alternatives to PHP’s mail()
function, along with code samples for each.
FAQ 1: How can I send an email in PHP using SMTP?
Answer: You can use PHPMailer, a popular PHP library for sending emails via SMTP.
require 'PHPMailerAutoload.php';
$mail = new PHPMailer;
$mail->isSMTP();
$mail->Host = 'smtp.example.com';
$mail->SMTPAuth = true;
$mail->Username = 'your_email@example.com';
$mail->Password = 'your_password';
$mail->SMTPSecure = 'tls';
$mail->Port = 587;
$mail->setFrom('from@example.com', 'Mailer');
$mail->addAddress('to@example.com', 'Receiver Name');
$mail->Subject = 'Here is the subject';
$mail->Body = 'This is the HTML message body <b>in bold!</b>';
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
if(!$mail->send()) {
echo 'Message could not be sent. Mailer Error: ' . $mail->ErrorInfo;
} else {
echo 'Message has been sent';
}
FAQ 2: How can I send an email with attachments in PHP?
Answer: You can send emails with attachments using PHPMailer.
$mail = new PHPMailer;
// ... SMTP configuration ...
$mail->setFrom('from@example.com', 'Mailer');
$mail->addAddress('to@example.com', 'Receiver Name');
$mail->Subject = 'Subject with Attachment';
$mail->Body = 'Email Body';
// Attach a file
$mail->addAttachment('/path/to/file.pdf');
if(!$mail->send()) {
echo 'Message could not be sent.';
} else {
echo 'Message has been sent with attachment';
}
FAQ 3: Is there a way to send emails asynchronously in PHP?
Answer: PHP itself does not support asynchronous operations natively. However, you can use a queue system like RabbitMQ or a job processing system like Gearman to process email sending asynchronously.
FAQ 4: Can I use external SMTP services like SendGrid or Mailgun to send emails from PHP?
Answer: You can integrate external SMTP services. Here’s an example with SendGrid.
require 'vendor/autoload.php';
$email = new \SendGrid\Mail\Mail();
$email->setFrom("test@example.com", "Example User");
$email->setSubject("Sending with SendGrid is Fun");
$email->addTo("test@example.com", "Example User");
$email->addContent("text/plain", "and easy to do anywhere, even with PHP");
$sendgrid = new \SendGrid('SENDGRID_API_KEY');
try {
$response = $sendgrid->send($email);
print $response->statusCode() . "\n";
print_r($response->headers());
print $response->body() . "\n";
} catch (Exception $e) {
echo 'Caught exception: '. $e->getMessage() ."\n";
}
FAQ 5: How can I ensure that my emails do not end up in the spam folder?
Answer: Ensuring emails do not end up in spam involves a few best practices:
- Use a reputable SMTP service.
- Ensure your domain has proper SPF, DKIM, and DMARC records.
- Avoid using spam trigger words in your email’s subject and body.
- Keep your sending reputation good by not sending too many emails too quickly.
Remember to replace placeholders in these code samples with your actual SMTP server details, email addresses, and other relevant information.
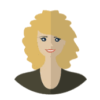
Ashley is an esteemed technical author specializing in scientific computer science. With a distinguished background as a developer and team manager at Deloit and Cognizant Group, they have showcased exceptional leadership skills and technical expertise in delivering successful projects.
As a technical author, Ashley remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Their expertise in PHP web development, coupled with their experience as a developer and team manager, positions them as a valuable resource for professionals seeking guidance and best practices. With each publication, Ashley strives to empower readers, inspire creativity, and propel the field of scientific computer science forward.