Understanding PHP’s Loosely Typed Nature” refers to comprehending how PHP, a popular scripting language used primarily for web development, manages variables and data types. In PHP, variables do not require explicit declaration of their data type.
This means the type of a variable is determined automatically based on the context or the value it is assigned. For example, a variable can be assigned a string and then reassigned a numeric value without error. While this offers flexibility and ease of use, it also requires careful handling to avoid unexpected behaviors or bugs in the code.
Tech CEOs should understand that this feature can impact both the development speed and the potential for errors in applications written in PHP.
What is a Loosely Typed Language?
A Loosely Typed Language is a programming language in which variables do not have a fixed type. In these languages, the type of a variable is determined at runtime and can change, allowing a variable initially holding a string, for example, to later store a number.
This approach offers flexibility and ease of development, as it requires less formal structure and fewer lines of code for data type management. However, it can also lead to errors or unpredictable behavior if not managed carefully, since the system might interpret the data type differently than expected.
Tech CEOs should understand that while loosely typed languages can accelerate development, they also require robust testing and error handling strategies to ensure application reliability.
Weak Typing in PHP
Understanding Weak Typing in PHP” means recognizing how PHP, a widely-used web scripting language, handles data types in a flexible, or ‘weak’, manner. In PHP, the type of a variable is determined by its context or the data it holds at any given moment.
This allows variables to be easily and dynamically converted between different types, such as from a string to a number, often implicitly. While this weak typing system simplifies coding and can speed up development, it also necessitates careful attention to avoid errors or unexpected behaviors in the program.
For tech CEOs, it’s important to understand that while weak typing in PHP enhances development agility, it also demands diligent testing and error handling to ensure the robustness and reliability of applications.
Dynamic Typing in PHP
Dynamic typing in PHP refers to the language’s ability to automatically determine the type of a variable based on the context or the value assigned to it at runtime.
This means that the same variable can hold different types of data over its lifetime without explicitly declaring its type.
Key Characteristics of Dynamic Typing in PHP:
- Type Flexibility: Variables can change types dynamically.
- Implicit Type Conversion: PHP automatically converts data types as needed.
- Ease of Use: Simplifies coding, especially for beginners.
- Potential for Errors: Requires careful handling to avoid type-related bugs.
Example Code Demonstrating Dynamic Typing in PHP:
<?php
// Initial assignment as an integer
$variable = 10;
echo gettype($variable); // Outputs: integer
// Reassigning the same variable as a string
$variable = "Hello, World!";
echo gettype($variable); // Outputs: string
// Using the variable as a boolean
$variable = true;
echo gettype($variable); // Outputs: boolean
?>
Comparison Table: Static vs Dynamic Typing in PHP
Aspect | Static Typing | Dynamic Typing in PHP |
---|---|---|
Type Declaration | Required at declaration | Not required |
Type Change | Not allowed after declaration | Allowed anytime |
Type Checking | At compile-time | At runtime |
Flexibility | Less flexible | More flexible |
Error Detection | Earlier (compile-time) | Later (runtime) |
For tech CEOs, understanding dynamic typing in PHP is crucial as it affects how quickly and efficiently your development team can build and maintain applications. It allows for rapid prototyping but requires a strong emphasis on testing to ensure code reliability.
Benefits of Loose Typing in PHP
Loose typing in PHP refers to the language’s flexibility in handling different data types, allowing for automatic type conversion based on the context. This feature can significantly enhance development efficiency but also requires careful consideration to avoid potential issues.
Benefits of Loose Typing in PHP:
- Development Speed: Quicker coding due to less need for explicit type declarations.
- Flexibility: Easy to manipulate variables for different purposes.
- Simplified Syntax: Less verbose code, making it more readable and maintainable.
- Ease of Use: Beginners find it more approachable to start coding.
Example Code Demonstrating Loose Typing in PHP:
<?php
// Assigning an integer to a variable
$number = 10;
// PHP automatically converts types
$total = "20" + $number; // String "20" is converted to an integer
echo $total; // Outputs: 30
// Using the same variable for different types
$number = "Ten";
echo $number; // Now it's a string
?>
Comparison Table: Loose vs Strict Typing
Aspect | Loose Typing in PHP | Strict Typing |
---|---|---|
Type Conversion | Automatic | Manual/Explicit |
Error Handling | Less strict, may lead to subtle bugs | More strict, errors caught early |
Code Verbosity | Less verbose, simpler code | More verbose, detailed declarations |
Learning Curve | Easier for beginners | Requires understanding of data types |
For tech CEOs, understanding the benefits of loose typing in PHP is important. It can lead to faster development cycles and easier code management, especially for projects with tight deadlines or smaller teams. However, it’s also essential to balance this with good coding practices and thorough testing to ensure software quality and reliability.
Challenges with Loosely Typed PHP
Loosely typed PHP offers flexibility, but it also comes with certain challenges that can affect code reliability and maintenance. Understanding these challenges is crucial for efficient management and decision-making regarding PHP projects.
Challenges with Loosely Typed PHP:
- Unpredictable Behavior: Automatic type conversion can lead to unexpected results.
- Debugging Difficulty: Identifying bugs related to type errors can be challenging.
- Performance Issues: Implicit type conversion can impact performance.
- Maintainability: Code readability and predictability can be compromised.
Example Code Demonstrating Challenges in Loosely Typed PHP:
<?php
$number1 = "10";
$number2 = 5;
// Implicit type conversion can lead to confusion
$result = $number1 + $number2; // Outputs 15, but $number1 is a string
echo $result;
// Potential for logical errors due to type juggling
if ("10" == 10) {
// This block will execute, might not be the intended behavior
echo "Equal";
}
?>
Comparison Table: Challenges in Loosely vs Strictly Typed Languages
Challenge | Loosely Typed PHP | Strictly Typed Languages |
---|---|---|
Type Safety | Lower, due to implicit conversions | Higher, with explicit declarations |
Bug Detection | Harder, especially for type-related bugs | Easier, as many errors are caught early |
Performance | Can be impacted by runtime type checking | Generally more optimized |
Code Predictability | Lower, due to dynamic type changes | Higher, with more predictable behavior |
For tech CEOs, it’s important to recognize that while loosely typed PHP accelerates development, it also necessitates stringent code reviews, testing, and potentially additional training for developers to handle these challenges effectively.
Balancing the flexibility of PHP with robust development practices is key to maintaining high-quality, reliable software.
Comparing PHP’s Loose Typing with Strongly Typed Languages
Comparing PHP’s loose typing with strongly typed languages involves understanding the fundamental differences in how these languages handle data types. PHP’s loose typing offers flexibility and ease of use, while strongly typed languages enforce strict data type rules, enhancing predictability and reducing certain types of errors.
Key Differences:
- Type Flexibility: PHP allows variables to change types dynamically, while strongly typed languages require explicit type declaration and conversion.
- Error Handling: PHP may hide certain type errors until runtime, whereas strongly typed languages often catch these errors during compilation.
- Development Speed: PHP’s loose typing can speed up development, especially in the initial stages, while strongly typed languages may require more upfront effort for type definitions.
Example Code:
PHP (Loosely Typed)
<?php
$var = "8";
$var += 2; // $var is now 10 (integer)
echo $var; // Outputs: 10
?>
Java (Strongly Typed)
public class Main {
public static void main(String[] args) {
String var = "8";
var += 2; // Error: cannot convert from int to String
System.out.println(var);
}
}
Comparison Table: PHP vs Strongly Typed Languages
Feature | PHP (Loosely Typed) | Strongly Typed Languages |
---|---|---|
Type Declaration | Not required | Mandatory |
Type Conversion | Implicit | Explicit |
Error Detection | At runtime | At compile-time |
Flexibility | High | Low |
Predictability | Lower | Higher |
For tech CEOs, understanding this comparison is crucial for making informed decisions about technology stacks. PHP’s loose typing can be beneficial for rapid development and prototyping, but strongly typed languages might offer better long-term maintainability and robustness, especially for large-scale or complex projects.
The choice depends on the project requirements, team expertise, and desired balance between development speed and code stability.
Best Practices for working with Loosely Typed PHP
Working with loosely typed PHP effectively requires adopting best practices to mitigate potential issues like unexpected behavior or hard-to-find bugs. Adhering to these practices can enhance code quality and maintainability.
Best Practices for Working with Loosely Typed PHP:
- Explicit Type Casting: Manually convert variable types when necessary to avoid ambiguity.
- Use Type-Checking Functions: Utilize functions like is_int(), is_string() for clarity.
- Strict Comparison Operators: Use === and !== to prevent unintended type juggling.
- Error Reporting and Logging: Enable comprehensive error reporting during development.
- Unit Testing: Implement tests to catch type-related bugs.
Example Code Demonstrating Best Practices:
<?php
// Explicit Type Casting
$var = "10";
$total = (int)$var + 5; // Explicitly cast string to integer
// Using Type-Checking Functions
if (is_string($var)) {
echo "It's a string";
}
// Strict Comparison Operators
if ($var === "10") {
echo "Exact match";
}
// Enabling Error Reporting
error_reporting(E_ALL);
ini_set("display_errors", 1);
// Unit Testing (Pseudo-code)
// testAddition("10", 5, 15); // Should fail, highlighting type issue
?>
Table: Best Practices in Loosely vs Strictly Typed Languages
Best Practice | Application in PHP | Application in Strictly Typed Languages |
---|---|---|
Type Casting | Often necessary | Rarely needed |
Type-Checking | Crucial for clarity | Less frequent, due to explicit types |
Comparison Operators | Use strict operators (=== , !== ) | Natural part of the language |
Error Handling | Essential due to runtime type issues | Compile-time checks reduce runtime errors |
Testing | Critical to catch type-related issues | Important, but fewer type-related concerns |
For tech CEOs, understanding these best practices is vital. It ensures that your teams are equipped to leverage PHP’s flexibility while maintaining high code quality. This approach reduces the risk of bugs and improves the reliability and scalability of your PHP-based applications.
Wrapping Up
Understanding PHP’s loose typing is essential for developers to write efficient and reliable code. PHP’s weak typing characteristics and dynamic typing make it a flexible and versatile language to work with, allowing for greater productivity and rapid prototyping. However, working with loosely typed PHP also presents challenges such as type coercion, potential errors caused by implicit type conversion, and the need for careful handling of data types.
We have discussed various aspects of PHP’s loosely typed nature, a characteristic that impacts how variables and data types are handled in PHP. This feature provides flexibility and speeds up development but also introduces challenges such as unpredictability and potential for errors.
Key points covered include:
- Dynamic Typing: PHP’s ability to assign and change variable types at runtime.
- Benefits of Loose Typing: Facilitates rapid development and code simplicity.
- Challenges: Includes issues like unpredictable behavior and debugging difficulty.
- Comparison with Strongly Typed Languages: Contrasts PHP’s type flexibility with the strict type enforcement of languages like Java or C#.
- Best Practices: Strategies like explicit type casting, strict comparison operators, and thorough testing to mitigate the risks associated with loose typing.
Each section provided code examples and comparison tables to illustrate the concepts, making it clear that while PHP’s loose typing offers development ease, it requires careful management to ensure code quality and reliability.
For tech CEOs, this knowledge is crucial for making informed decisions about using PHP in their projects, balancing its flexibility with robust development practices to achieve efficient and stable software solutions.
External Resources
https://en.wikipedia.org/wiki/PHP
https://www.w3schools.com/php/
FAQ
What is loosely typed nature in PHP?
PHP doesn’t require explicit declaration of variable types. The type is inferred from the context or the value assigned.
Example:
$var = "Hello"; // $var is a string
$var = 10; // Now, $var is an integer
How does PHP handle type conversion?
PHP automatically converts types as needed, which can lead to unexpected results if not carefully managed.
Example:
$a = "5" + 10; // String "5" is converted to integer
echo $a; // Outputs: 15
What are the risks of PHP’s loose typing?
It can lead to subtle bugs due to implicit type conversions and may cause unexpected behavior in logical operations.
Example:
if ("10" == 10) {
echo "Equal"; // This will output "Equal" due to type juggling
}
How can I ensure type safety in PHP?
Use strict comparison operators (===, !==) and explicit type casting when necessary.
Example:
if ("10" === 10) {
echo "Equal";
} else {
echo "Not Equal"; // This will output "Not Equal"
}
What are the best practices for working with PHP’s loosely typed nature?
Employ explicit type casting, use type-checking functions, and apply strict comparison operators to avoid unexpected behavior.
Example:
$num = "5";
$num = (int)$num; // Explicit type casting
if (is_int($num)) {
echo "Integer"; // Outputs: Integer
}
These questions and code samples highlight the key aspects of PHP’s loosely typed nature, demonstrating both its flexibility and the importance of understanding its implications for reliable coding practices.
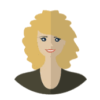
Ashley is an esteemed technical author specializing in scientific computer science. With a distinguished background as a developer and team manager at Deloit and Cognizant Group, they have showcased exceptional leadership skills and technical expertise in delivering successful projects.
As a technical author, Ashley remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Their expertise in PHP web development, coupled with their experience as a developer and team manager, positions them as a valuable resource for professionals seeking guidance and best practices. With each publication, Ashley strives to empower readers, inspire creativity, and propel the field of scientific computer science forward.