Are you a WordPress developer looking to improve your PHP coding skills? Look no further than this comprehensive guide to WordPress PHP best practices. By following these practices, you can optimize your coding, improve site performance, and enhance site security.
When coding in PHP for WordPress, it is essential to understand the importance of optimized and efficient code.
PHP in the WordPress Environment
PHP is a crucial component of the WordPress environment. It is the backbone of theme and plugin development, as well as custom functionality implementation. PHP code is executed on the server-side, which powers all the dynamic content of a WordPress site, including page templates, custom post types, and user-generated content.
WordPress comes with its own set of PHP functions and classes, such as WP_Query and get_sidebar(), to simplify common tasks and ensure consistency. Additionally, WordPress uses hooks and filters to allow developers to modify and extend core features without modifying the original code. Understanding these functions and hooks is essential to efficiently develop with PHP in the WordPress environment.
Because WordPress is written in PHP, it is important for developers to have a solid understanding of the language. Knowledge of PHP data types, operators, functions, and control structures is necessary to build efficient and maintainable WordPress applications.
Clean and Efficient Code
When writing PHP code in WordPress, it is essential to optimize your coding for performance and efficiency. Here are some tips for optimizing PHP coding in WordPress:
- Minimize the use of global variables: Using global variables can slow down your code and create potential conflicts with other functions. When possible, use local variables instead to improve performance.
- Avoid excessive database queries: Every time you make a database query, there is a performance overhead. Instead of making multiple queries, consider using a single query with appropriate JOINs or a more efficient query technique.
- Optimize loops: Loops can also be a performance bottleneck. Consider using efficient loop techniques like foreach, map or reduce.
By optimizing your PHP code in WordPress, you can boost your site’s performance, improve its usability, and even enhance its security.
Following WordPress PHP Coding Standards
Adhering to WordPress PHP coding standards is imperative for creating clean, readable, and maintainable code that is universally compatible with all versions of WordPress. Strict adherence to coding standards improves the overall quality of your code, and provides an excellent foundation for collaboration with other developers. These standards cover every aspect of PHP coding, including variable naming conventions, function structures, and formatting guidelines.
By following coding standards, you ensure that your code is consistent and easy to follow, making it more accessible to a wider audience. The WordPress community has developed an extensive list of coding standards, which are updated on a regular basis to ensure optimal compatibility with future versions. Staying current with these standards is an essential component of any WordPress development project.
Examples of WordPress PHP Coding Standards
Here are few examples of WordPress PHP coding standards:
Coding Standard | Explanation |
---|---|
Use “camelCase” for function names | This standard dictates that function names should be in “camelCase”. For instance, “myFunctionName”. |
Use “snake_case” for variable names | This standard dictates that all variable names should be in “snake_case”. For instance, “$my_variable_name”. |
Use spaces after operators | This standard dictates that you should use spaces after operators, such as ‘=’, ‘+’, ‘-‘, etc. |
Adopting these standards when coding your WordPress PHP functions and classes will ensure that your code is consistent with the standards of the broader community. It will also make your development work more accessible to other developers who are familiar with coding standards, making it easier for your code to be reviewed and improved upon.
Properly Using WordPress APIs and Functions
Utilizing WordPress APIs and functions is essential for effectively developing themes, plugins, and custom functionality in WordPress. These built-in features make it easy to accomplish common tasks, interact with data, and manipulate content. Below are some key considerations when working with WordPress APIs and functions.
Understanding WordPress Core Functions
The WordPress core provides a vast collection of functions that developers can harness to add new features to WordPress. Some of the most commonly used functions include:
Function | Description |
---|---|
get_header() | Retrieves the header template for a theme or a specific header template file if one is specified. |
the_content() | Displays the post content. |
get_post() | Retrieves a specific post object by ID. |
add_action() | Links a specific function to a specific action hook. |
These functions, along with many others, can be combined and customized to create powerful, dynamic functionality in WordPress. Knowing how to leverage these core functions is a key aspect of becoming an efficient WordPress developer.
Understanding WordPress APIs
WordPress also provides several APIs that developers can use for more specialized functionality. These include:
- The Options API, for handling site options and settings
- The Settings API, for creating settings pages and forms
- The Shortcode API, for creating custom shortcodes to display content
- The HTTP API, for making HTTP requests to external resources
By utilizing these APIs, developers can extend WordPress in powerful ways while maintaining compatibility and adhering to best practices.
Benefits of Using WordPress APIs and Functions
By using WordPress APIs and functions, developers can take advantage of built-in functionality without the need to write extensive custom code. This can save significant development time and help maintain compatibility with future WordPress updates. Additionally, utilizing WordPress APIs and functions can help ensure that your code remains secure, efficient, and easy to maintain.
Securing Your WordPress PHP Code
When it comes to securing PHP code in WordPress, there are several measures that you can take to protect your website against malicious attacks.
First and foremost, always validate user input. This is crucial in preventing SQL injection attacks, which can compromise your WordPress site’s database. Use WordPress’s built-in functions, such as esc_html() and esc_attr(), to sanitize user input and prevent these types of attacks.
Another important security measure is to properly handle files in your PHP code. Be cautious of file uploads and always validate the file type and size before storing it on your server. Use the WordPress function wp_handle_upload() to handle file uploads and ensure that they are secure. Additionally, ensure that file permissions are set properly to prevent unauthorized access.
It’s also important to safeguard against cross-site scripting attacks (XSS) by using the appropriate WordPress functions for outputting data, such as esc_html_e() and esc_attr_e(). These functions automatically sanitize the output and prevent attackers from inserting malicious code into your website.
Finally, be aware of common security vulnerabilities in WordPress plugins and themes. Regularly update your plugins and themes to ensure that any security issues are patched, and always use trusted sources for downloading new plugins and themes.
By following these best practices for securing PHP code in WordPress, you can help protect your website against malicious attacks and ensure the safety of your site and its users.
Utilizing Caching and Optimization Techniques
Optimizing PHP code in WordPress is vital to improve site performance. Caching techniques in WordPress can help reduce the number of database queries and speed up page load times. There are several optimization techniques you can implement to improve the performance of your PHP code in WordPress.
Caching Queries
One of the most effective ways to speed up your WordPress website is by caching queries. Caching involves storing query results in memory so that subsequent requests for the same data can be returned faster. WordPress provides a built-in caching system that you can use to cache database queries.
To cache a query result, you can use the wp_cache_get() and wp_cache_set() functions provided by WordPress. These functions allow you to store the result of a query in the cache and retrieve it from the cache on subsequent requests.
For example:
<?php
$result = wp_cache_get( 'my_query', 'my_group' );
if ( false === $result ) {
// Query the database
// Store the result in the cache
$result = /* Query result */;
wp_cache_set( 'my_query', $result, 'my_group' );
}
// Use the query result
echo $result;
?>
Using Transients
Another caching technique you can use in WordPress is transients. Transients allow you to store temporary data in the database for a specified amount of time.
To use transients, you can use the set_transient() and get_transient() functions provided by WordPress. These functions allow you to store and retrieve transient data easily.
For example:
<?php
$result = get_transient( 'my_transient' );
if ( false === $result ) {
// Query the database
// Store the result in the transient
$result = /* Query result */;
set_transient( 'my_transient', $result, 3600 ); // Expires in 1 hour
}
// Use the query result
echo $result;
?>
Optimizing Assets
Optimizing assets can also help improve site performance. You can optimize assets such as images, scripts, and stylesheets by reducing their file size and minimizing the number of requests to the server.
To compress images, you can use a tool like Photoshop or an online image optimizer. To reduce the size of scripts and stylesheets, you can use a tool like Minify or GZIP compression.
Another technique for optimizing assets is to use a content delivery network (CDN). A CDN works by caching your website’s assets on servers located around the world, reducing the distance between the user and the server and improving page load times.
By implementing these caching and optimization techniques, you can greatly improve the performance of your PHP code in WordPress and provide a better user experience for your visitors.
Debugging and Error Handling
Even with careful coding and adherence to best practices, errors can still occur in PHP code for WordPress. It’s important to have effective debugging and error handling techniques in place to quickly identify and resolve these issues.
WordPress provides a variety of built-in debugging tools to assist with error identification and resolution. These include the debug log, which can be enabled in the wp-config.php file, as well as the WP_DEBUG constant, which when set to true, displays error messages on the site. It’s also possible to use third-party debugging tools such as Xdebug for more advanced debugging.
When handling errors in PHP code for WordPress, it’s important to use proper error handling techniques to prevent potentially sensitive information from being exposed to end users. This includes using the try-catch block to catch and handle errors, and properly sanitizing user input to prevent SQL injection and other vulnerabilities.
Common PHP errors in WordPress include undefined variables, syntax errors, and white screen of death (WSOD) errors. By utilizing WordPress debugging functions and error handling techniques, these issues can be quickly resolved and site functionality can be restored.
Working with Themes and Plugins
WordPress themes and plugins are the building blocks of your website’s design and functionality. As a PHP developer, it’s crucial to understand the basics of PHP coding in WordPress themes and plugins to be able to create custom functionality and tailor designs to your specific needs.
PHP Coding in WordPress Themes
WordPress themes are responsible for the appearance and layout of your website. When creating a custom WordPress theme, it’s important to follow best practices for PHP coding to ensure compatibility with other plugins and themes.
One of the key aspects of PHP coding in WordPress themes is understanding the template hierarchy. The template hierarchy defines the order in which WordPress loads different template files to render a specific page. By following the hierarchy, you can ensure that your theme is compatible with other plugins and themes that may be installed on the site.
Another important aspect of PHP coding in WordPress themes is utilizing actions and filters. WordPress has a vast number of hooks that allow developers to modify or add to the functionality of WordPress without having to modify core code. By utilizing these hooks, you can create a more flexible and customizable theme.
PHP Coding in WordPress Plugins
WordPress plugins add extra functionality to your website that is not included in the core WordPress software. When developing a custom plugin, it’s important to follow best practices for PHP coding to ensure compatibility with other themes and plugins.
One important aspect of PHP coding in WordPress plugins is understanding how to use actions and filters. By using these hooks, you can modify or add to the functionality of WordPress core code without having to modify the core directly.
Another important aspect of PHP coding in WordPress plugins is adhering to WordPress PHP coding standards. By following these standards, you can ensure that your plugin is compatible with future updates of WordPress and other plugins.
Whether creating a theme or plugin, following best practices for PHP coding in WordPress is essential to ensure compatibility, security, and maintainability of your code.
Managing WordPress Updates and Compatibility
Keeping WordPress up-to-date is vital for maintaining your site’s functionality and security. However, updating WordPress, themes, and plugins can sometimes cause compatibility issues with your PHP code
One best practice is testing your PHP code on a staging site before updating your live site. This is particularly important if you’ve made customizations to your theme or plugin. Version control is another best practice. It will help you manage code changes and rollback to a previous version quickly if necessary.
When it comes to updating WordPress, themes, and plugins, plan for updates ahead of time. Check your theme and plugin documentation to see if they are compatible with the latest version of WordPress. If an update is available, make sure to backup your site before updating.
After updates, always check your site’s functionality and compatibility with your PHP code. Look for error messages and inconsistencies in the layout and functionality of your site. If you notice anything, consider troubleshooting or seeking support from the relevant vendor or community.
Keeping your PHP code up to date with WordPress updates requires consistent effort, but it is necessary to ensure your site’s performance and security. Follow these best practices to avoid unwanted headaches and maintain the stability of your site.
Performance Optimization Techniques
Optimizing PHP code in WordPress is crucial for site performance. By reducing database queries, optimizing loops, caching results and using asynchronous processes, you can improve both page load times and user experience. Here are some techniques to help:
Reducing Database Queries
Each database query takes time, so reducing the number of queries can significantly improve performance. One way to achieve this is by using caching plugins. Another option is to use WordPress functions like get_posts() and WP_Query() to retrieve multiple posts at once instead of using multiple queries.
Optimizing Loops
Loops can be resource-intensive, especially if they involve many data queries. To optimize loops, use WordPress functions like get_the_ID() and get_the_title() to avoid unnecessary queries. Additionally, try to minimize code inside loops and use proper array functions such as array_map() and array_filter() to avoid using for loops when possible.
Caching Results
Caching allows WordPress to store resources in memory or disk, reducing the need to fetch them from the server each time they are requested. WordPress provides multiple caching methods such as Transients API, Object Cache, and HTTP caching through web servers like Apache and NGINX.
Using Asynchronous Processes
Asynchronous processes allow PHP code in WordPress to run independently of the main application thread. This can significantly improve performance for resource-intensive tasks without affecting user experience. WordPress provides WP-Cron, a built-in job scheduler that allows developers to schedule tasks to run at specific intervals or times.
Conclusion
As a WordPress developer, following best practices when coding in PHP is paramount to building high-quality, secure, and performant websites. By implementing the tips and techniques discussed in this comprehensive guide, you can optimize your code, improve site performance, and enhance site security.
Adhering to WordPress PHP coding standards ensures that your code is maintainable and compatible with future updates, while proper utilization of WordPress APIs and functions streamlines common tasks. Securing your PHP code protects against vulnerabilities and utilizing caching and optimization techniques improves performance.
Effective debugging and error handling ensures a smoother development process, and following best practices for PHP coding in themes, plugins, and custom functionality is essential. Managing updates and compatibility ensures code quality remains high, and performance optimization techniques reduce database queries, optimize loops, and use asynchronous processes.
By implementing these best practices, you can ensure your WordPress PHP code is of the highest quality and your website’s performance and security are optimized.
External Resources
https://en-gb.wordpress.org/plugins/
FAQ
Q: What are WordPress PHP best practices?
A: WordPress PHP best practices are guidelines and techniques that developers follow to optimize their PHP code in the WordPress environment. These practices help improve performance, enhance security, and ensure compatibility with future updates.
Q: How is PHP used in the WordPress environment?
A: PHP is a scripting language used to create dynamic web pages. In the WordPress environment, PHP is used extensively to develop themes, plugins, and custom functionality. It is integrated with WordPress functions and hooks to interact with the WordPress core and modify its behavior.
Q: Why is clean and efficient code important in PHP for WordPress?
A: Writing clean and efficient code is crucial in PHP for WordPress as it improves site performance, enhances maintainability, and makes future development easier. Optimizing PHP code involves techniques like reducing the use of global variables, minimizing database queries, and optimizing loops.
Q: What are WordPress PHP coding standards?
A: WordPress PHP coding standards are a set of guidelines and conventions that developers follow when writing PHP code for WordPress. Adhering to these standards ensures code readability, maintainability, and compatibility with the WordPress ecosystem and future updates.
Q: Why should I utilize WordPress APIs and functions?
A: Utilizing WordPress APIs and functions is essential to accomplish common tasks efficiently and securely. WordPress provides a rich set of APIs and functions for retrieving post data, querying the database, handling user authentication, and more. By leveraging these core functions, developers can avoid reinventing the wheel and ensure compatibility with future updates.
Q: How can I secure my PHP code in WordPress?
A: Securing PHP code in WordPress involves implementing measures like input validation, data sanitization, secure file handling, and protecting against common security vulnerabilities. Following security best practices helps safeguard your code and protect your site from malicious attacks.
Q: What are caching and optimization techniques in PHP code for WordPress?
A: Caching and optimization techniques in PHP code for WordPress aim to improve site performance by reducing server load and speeding up page load times. Techniques include caching queries, using transients, and optimizing assets. These optimizations can have a significant impact on the overall user experience.
Q: How can I effectively debug and handle errors in PHP for WordPress?
A: Debugging and error handling in PHP for WordPress involve using WordPress debugging tools, enabling error logging, and troubleshooting common PHP errors. Implementing robust debugging and error handling techniques helps identify and resolve issues quickly, ensuring a smooth user experience.
Q: What should I consider when working with themes and plugins in PHP for WordPress?
A: When working with themes and plugins in PHP for WordPress, it’s important to follow best practices like maintaining a proper theme structure, understanding the template hierarchy, and utilizing hooks and filters for extending functionality. These practices ensure code organization, reusability, and compatibility.
Q: How do I manage WordPress updates and maintain PHP code compatibility?
A: Managing WordPress updates and maintaining PHP code compatibility requires strategies such as versioning code, performing compatibility testing, and staying updated with WordPress core changes. Outdated code can introduce security vulnerabilities and impact site performance, so it’s crucial to handle updates effectively.
Q: What are performance optimization techniques in PHP for WordPress?
A: Performance optimization techniques in PHP for WordPress involve reducing database queries, optimizing loops, caching results, and utilizing asynchronous processes. These techniques help improve site speed and responsiveness, creating a better user experience.
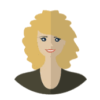
Ashley is an esteemed technical author specializing in scientific computer science. With a distinguished background as a developer and team manager at Deloit and Cognizant Group, they have showcased exceptional leadership skills and technical expertise in delivering successful projects.
As a technical author, Ashley remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Their expertise in PHP web development, coupled with their experience as a developer and team manager, positions them as a valuable resource for professionals seeking guidance and best practices. With each publication, Ashley strives to empower readers, inspire creativity, and propel the field of scientific computer science forward.